Learning Golang
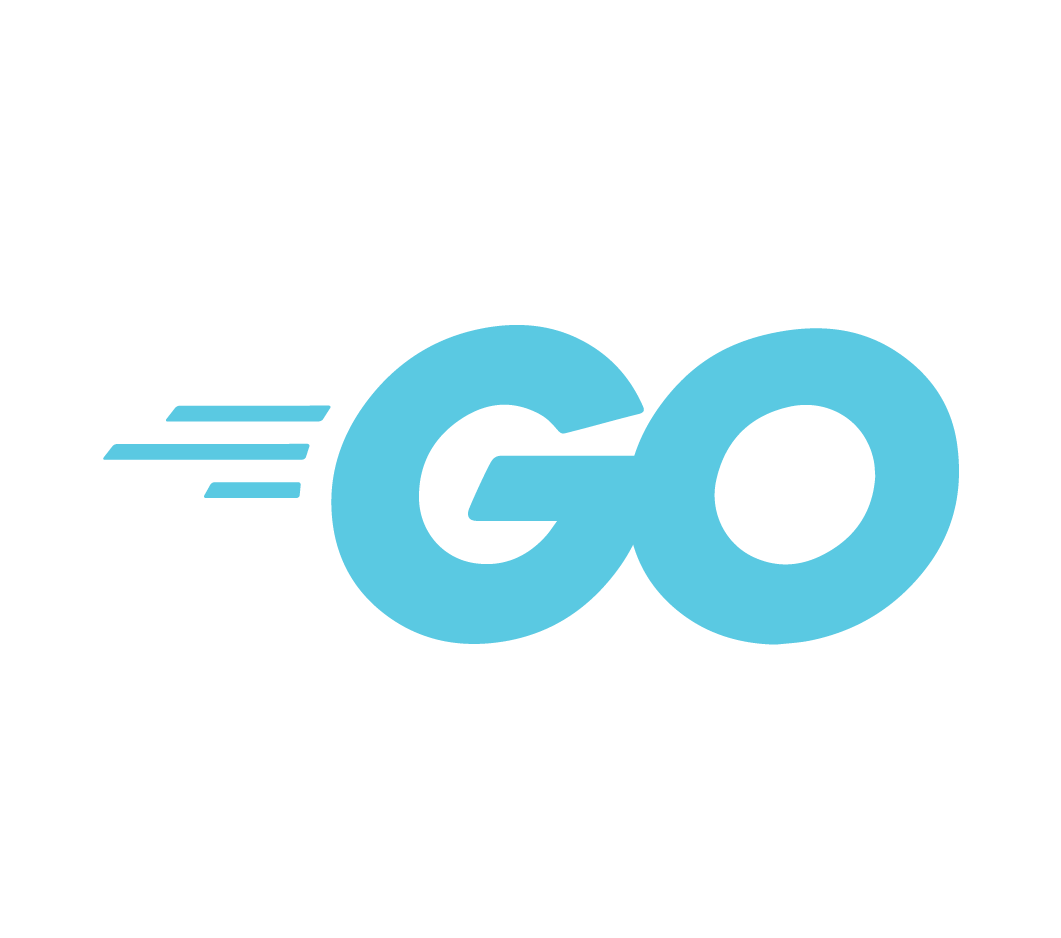
What's Go Lang for?
Introduction to Go video
Go is for
- Scale
- Large distributed systems, connecting thousands of machines
- The complexity of modern, interconected systems
- Supports:
- Interfaces
- Reflection
- Concurrency
- Assumes utf8 encoding throughout
Writing & Running Go Language Files
A basic Go language program must start with package main
followed (usually) by a package import and a func main()
declaration:
package main
import "fmt"
func main() {
fmt.Printf("Hello world")
}
Go is a compiled language, not an interpreted language like Python.
To compile and run an Go program write:
go run <filename>
e.g.
go run helloworld.go
Importing packages in Go
Single imports:
import "fmt"
Multiple imports
import (
"fmt"
"time"
)
You can define methods on any data representation
What?
This means that, for example, in Go you can define methods on ints. This isn't easily done or possible in other languages (like C), although possible in python with subclassing. The difference is that this capability is more 'built-in' in Go.
In python:
class myInt(int):
def __new__(cls, *args, **kwards):
return super(myInt, cls).__new__(cls)
def mymethod(self):
print "yolo"
How to subclass built-in imutable types in python. (above)
new() is intended mainly to allow subclasses of immutable types (like int, str, or tuple) to customize instance creation. It is also commonly overridden in custom metaclasses in order to customize class creation. See python Data model docs
The same in Go lang:
This takes a type Office
which is declared as an int
, but then adds a string method to the Office
type. Example its inspired by the introduction to Go video.
package main
import "fmt"
var officePlace[2]string
type Office int
const (
Boston Office = iota
NewYork
)
func (o Office) String() string {
return "Google, " + officePlace[o]
}
func main() {
officePlace[0] = "Cambridge, MA"
fmt.Printf("Hello, %s\n", Boston)
}
Adding a string method to type int
in Go lang.
The above
What is iota in Go?
An iota in Go is similar to an enum
in C, for example it's used in Golang's time
package to number the days of the week:
// A Weekday specifies a day of the week (Sunday = 0, ...).
type Weekday int
const (
Sunday Weekday = iota
Monday
Tuesday
Wednesday
Thursday
Friday
Saturday
)
See GoLang time weekday sourcecode
Printing time to fetch webpage in Go
This is a completed example of the "Tour of Go" talk which shows a snippet of how to log the duration of time it takes to fetch a webpage, written in Go. You'll notice the number of packages required grows, showing the growing amount of built-in packages for go:
package main
import (
"time"
"fmt"
"net/http"
"io"
"io/ioutil"
"log"
)
func fetch(url string) {
r, err := http.Get(url)
if err != nil {
log.Fatal(err)
}
io.Copy(ioutil.Discard, r.Body)
r.Body.Close()
}
func main() {
start := time.Now()
fetch("https://www.google.com/")
fmt.Println(time.Since(start))
}
Writing bytestreams in Go
Writing to a file in Go using os
and fmt.Fprintf
package. Note that these are all Writers.
package main
import (
"fmt"
"os"
"log"
)
func main() {
file, err := os.OpenFile("text.txt", os.O_WRONLY|os.O_CREATE, 0755)
if err != nil {
log.Fatal(err)
}
// This writes to stdout (the screen, not a file)
fmt.Fprintf(os.Stdout, "Hello, world!\n")
// This write to a file called "text.txt"
fmt.Fprintf(file, "Hello, world!\n")
// Close the file
if err := file.Close(); err != nil {
log.Fatal(err)
}
}